§ Бамп тред
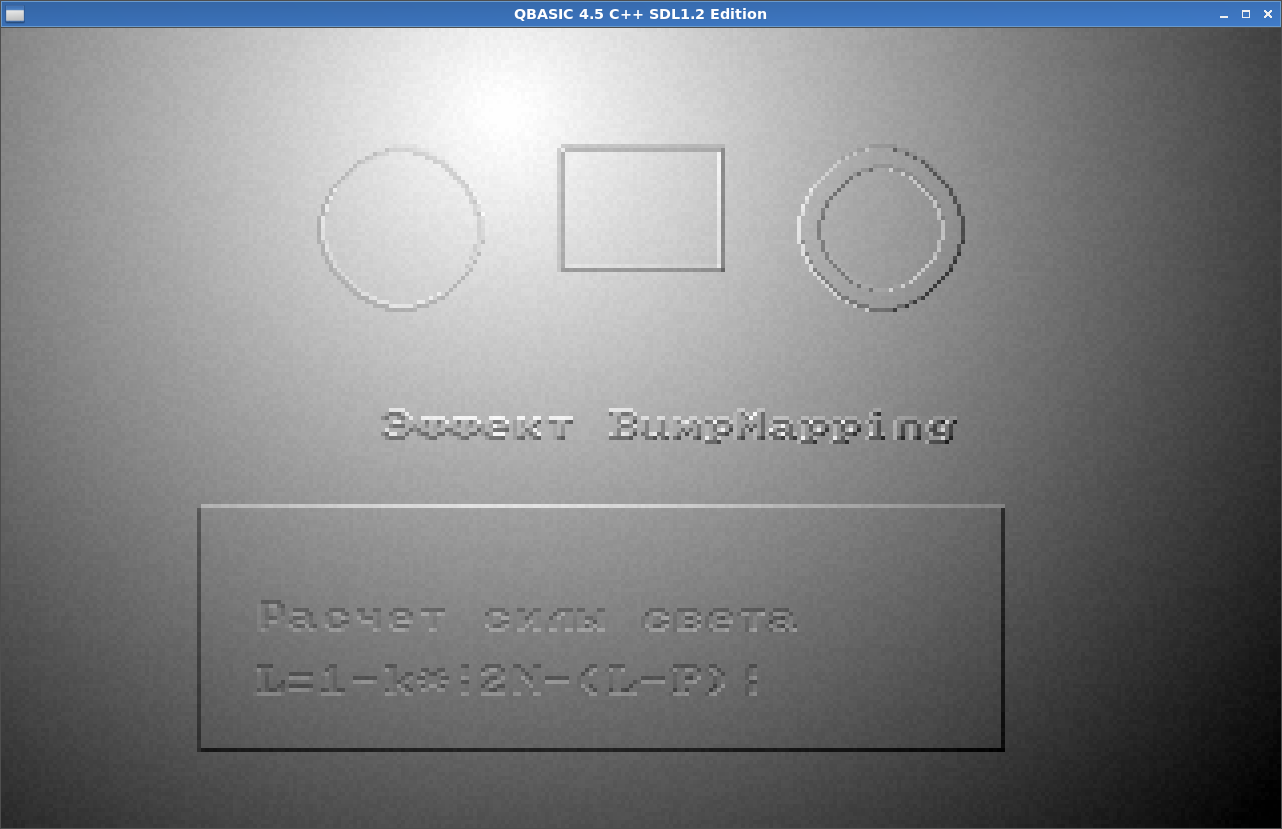
1#include <qblib.c>
2
3vec2 normal[320][200];
4int height[320][200];
5
6void calcnormal(int x, int y) {
7
8 normal[x][y].x = height[x][y] - height[(x+1)%320][y];
9 normal[x][y].y = height[x][y] - height[x][(y+1)%200];
10}
11
12void init() {
13
14 color(32, 0); cls();
15 locate(12, 12); print("Эффект BumpMapping");
16 circle(100, 50, 20, 16);
17 circlef(220, 50, 20, 32);
18 circlef(220, 50, 15, 8);
19 lineb(140, 30, 180, 60, 32);
20
21 linebf(50, 120, 250, 180, 32);
22 color(16, -1);
23 locate(8, 18); print("Расчет силы света");
24 locate(8, 20); print("L=1-k*|2N-(L-P)|");
25}
26
27int main(int argc, char* argv[]) {
28
29 screen(13);
30 init();
31
32 for (int i = 1; i < 256; i++) palette(i, i, i, i);
33 palette(255, 0, 255, 0);
34
35
36 for (int y = 0; y < 200; y++)
37 for (int x = 0; x < 320; x++)
38 height[x][y] = qb_screen[x][y];
39
40 for (int y = 0; y < 200; y++)
41 for (int x = 0; x < 320; x++)
42 calcnormal(x, y);
43
44 color(255, 0);
45 cls();
46
47 float t = 0;
48 while (sdlevent(EVT_REDRAW)) {
49
50 vec2 light = {160 + sin(t)*160, 100 + cos(3*t)*100};
51 t += 0.01;
52
53 srand(1);
54 for (int y = 0; y < 200; y++)
55 for (int x = 0; x < 320; x++) {
56
57 vec2 h = {
58 2*normal[x][y].x - (light.x - x),
59 2*normal[x][y].y - (light.y - y),
60 };
61
62
63 float l = (256 - sqrt(h.x*h.x + h.y*h.y));
64
65
66 l += rand()%7;
67
68
69 if (l < 1) l = 1;
70 else if (l > 254) l = 254;
71
72 pset(x, y, l);
73 }
74 }
75
76 return 0;
77}